Draw an image using the HTML <canvas> Tag
<h2>Original Image</h2>
<img id="glacier" src="http://www.htmlcodes.ws/images/examples/3s.jpg" alt="Photo of Rob Roy Glacier">
<h2>Canvas Images</h2>
<canvas id="myCanvas" width="350" height="220">
<p>Fallback content for browsers that don't support the canvas tag.</p>
</canvas>
<script>
var c = document.getElementById("myCanvas");
var ctx = c.getContext("2d");
// Original size
ctx.drawImage(glacier,5,25);
// Resized
ctx.drawImage(glacier,180,0,100,75);
// Clipped
ctx.drawImage(glacier,20,35,85,70,190,130,85,70);
</script>
Original Image
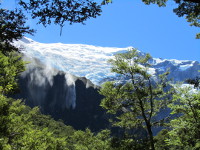
Canvas Images
The above example demonstrates how to draw an image using the HTML <canvas>
element.
Here, we add the source image as we would any other image (by using the <img>
tag). Now we can write that image to the <canvas>
by referring to the image's id
attribute value.
First, we draw the image to the canvas using the original dimensions of the source image. We then draw the image again, but this time, we resize it by specifying the width and height dimensions. The third canvas image has been clipped from the original, then that clipping has been drawn to the canvas in the size that we specify.
The drawImage()
Method
Drawing an image to a <canvas>
element, is done using the drawImage()
method. This method can be invoked with three different sets of arguments.
drawImage(image, dx, dy)
- This is
drawImage()
at its simplest. Theimage
parameter is used to specify the image. It can refer to an<img>
,<video>
or<canvas>
element.The
dx
argument is used to specify the horizontal coordinates of the destination canvas image. Thedy
represents the vertical coordinates. drawImage(image, dx, dy, dw, dh)
- You can add
dw
to specify the width of the destination canvas image, anddh
to specify the height. drawImage(image, sx, sy, sw, sh, dx, dy, dw, dh)
- This is used for clipping a part of the source image and drawing that to the canvas. The
sx
,sy
,sw
, andsh
parameters allow you to specify which part of the source image to clip. Thedx
,dy
,dw
, anddh
parameters allow you to specify where the result of that clipping will appear on the canvas, as well as its dimensions.